Are You a Developer?
VBG offers many options for developers to integrate voice biometrics into their applications.
You Choose the Development Approach
As we point out on our Integration Examples page, VBG offers several options for developers. So, you can choose whichever option best suits your style, or availability of resources, or particular use case. Clients having multiple applications can mix and match techniques too -- the choice is yours. Fundamentally, there are 3 basic approaches developers can take:
-
Custom Integration. With this option, developers have full control over their audio, and they use a lower-level API to integrate with the VBG Platform when and as needed. For instance, you can use our RESTful API to POST audio samples to the VBG Platform from your IVR system.
-
User Interaction Option. With this option, developers might use VBG's Standard Dialogs and a platform plug-in to greatly reduce development effort. For instance, using VBG's standard Enroll and Verify dialogs with our Webhook interfaces to Twilio, or Telnyx, or Aspect, or any of the other IVR and Call Center platforms we support.
-
Standard Plug-In. With this option, you can use a plug-in or adapter to enable voice biometric authentication, with little or no development needed. For instance, you can use our RADIUS Server plug-in to implement Multi-Factor Authentication for remote VPN users, with no development needed!
Next, we'll cover the basic requirements for developing against the VBG Platform. Then, we'll provide some background on the object model we use. And finally, we'll provide a high-level example for each of the integration steps we've outlined above. Should you have any questions after reading this section, please feel free to contact us.
VBG Platform Development Requirements
The VBG Platform needs speech samples in order to perform voice biometric processing and analysis. So, there are a few minimum requirements for developers to be aware of:
-
Speech Capture. Your application needs the ability to capture speech from the end user. Whether IVR system, or caller's leg of a call center conversation, or mobile phone, or headset, or IoT device -- we need a sample of the user's speech. And it's important to note that multiple speakers cannot be present in the sample.
-
Audio Format. Captured speech needs to be sent to us either as a recording, or via media streaming. Note that we currently work with RTP and Web Socket streams. Audio recordings need to be in WAV format as follows:
- a-Law, u-Law, or PCM WAV format
- 8K or 16K, 16-bit, mono
-
HTTPS Support. You must have the ability to communicate over HTTP/HTTPS with a 3rd party platform via API. We currently support REST, SOAP, VoiceXML, and Streaming. The 3rd party platform in this case is the VBG Platform.
Depending on what development approach you take, some or all of these requirements might be addressed for you. For instance, if you use one of VBG's platform (webhook) integrations and our Standard Dialogs, the audio capture, proper formatting, and speech sample transmission are all taken care of!
Key Objects to Know
Customer. Within the VBG Platform, "customer" is a unique application with respect to language and use case. For instance, our RandomPIN™ use case in English could be one customer. While our Static Passphrase use case in Spanish could be another. Our clients can (and typically) do have multiple customer definitions they access. Each customer typically has multiple users (userids) associated with it.
Userid. Within the VBG Platform, "userid" is a unique entry within a customer for an end user of the system. It is used to reference voiceprint storage, usage statistics, and other key metrics. There can be only one voiceprint per userid (user). However, the same userid can appear within different customers.
Sample. Within the VBG Platform, "sample" is a single audio recording of a userid (user) speaking. Samples are submitted to the voice biometric engine for processing -- to create a voiceprint (called "enrollment"), or to score against an existing voiceprint (called "verification" or "identification").
Transaction. Within the VBG Platform, "transaction" can best be thought of a container for one or more samples that are gathered during one session. So, if you imagine a phone call, and an end user submitting multiple speech samples during that phone call, we use a transaction to hold all the related samples from the call. Transactions always have one unique customer and one unique userid associated with them.
There are of course other objects in our data model. But for now, the above definitions will help us to work through some simple, high-level integration scenarios.
Setup for Examples
It's beyond the scope of this article to provide detailed, step-by-step development instructions for all possile scenarios. VBG has plenty of online documentation, examples, and code samples for developers when the time is right. For now, we'll make some simple assumptions and then consider some different development approaches.
First, we next to setup a customer in the VBG Platform. Let's call this customer xyzcompany-rn-eng (where "rn" is for our RandomPIN™ use case and "eng" is for "U.S. English"), and we'll assign an API access key 4659472b70a9dd75e80556a8efc3800f for security too. Note that VBG sets up all customers and API access keys as part of its security and provisioning process.
Next, let's setup a userid for the xyzcompany-rn-eng customer called myuser01. Using CURL and our RESTful API, it would look something like this:
HTTP/1.1 200 OK
Note that parameters such as “-d”, “-G”, and “-X POST” are syntax specific to CURL and are not relevant for any other development environment.
Now that we have a customer and a userid defined in the system, we'll look at the three different integration approaches using the most common voice biometric mode, verification (also referred to as authentication).
Verification is the process of comparing a speech sample for a user to the stored voiceprint for that same user, in order to obtain a similarity score. This score is then interpreted as either "passing" or "failing" and can then used to make a decision for the user.
Custom Integration Example
For this example, we'll assume you have your own IVR system, that myuser01 has called in, and that you will be using the VBG Platform to prompt myuser01 to repeat a random digit phrase in order to verify their identity using voice biometrics. Here is the summary of the steps you'll take:
-
Use GET for /users/{userid} API method to make sure userid exists and is active
-
Use POST for /transactions API method to create a verification transaction. Response is then parsed for important information your application needs.
-
Use POST for /samples API method to submit speech sample to the open verification transaction. Response is parsed to determine next steps.
-
Use GET for /transactions/{transactionid} API method to get information about the verification process, along with other key measures. This is a highly recommended step, but we won't cover it in much detail in this article.
-
Use POST for /transactions/{transactionid} API method to complete the transaction, making sure to note the final score received
Step 1 - Check Userid
Whenever a user identifies themselves to your system, it's a best practice to determine their status. Continuing on with our example scenario, here is a simple request in CURL:
The VBG Platform can respond in either XML or JSON format. An example JSON response is as follows:
Your application would parse this response. And as we can see from the highlighted values above, myuser01 is active in the system and has a model (a voiceprint) created. So, your application now knows it can proceed ahead with a verification request when needed.
Step 2 - Create Verification Transaction
Next, since you know myuser01 can be verified, you would create a verification transaction for them (prior to starting the prompting process within your IVR system). An example request using CURL follows:
As you can see from the CURL request, the parameters for userid and type and calltype were provided. An example JSON response is as follows:
Again, your application would parse this response. Please note that the Transaction object is extremely flexible, covering many enrollment and verification scenarios. So, there are many potential values. For now, the most important two values are highlighted: (1) "id" which is the transaction id, and (2) "prompt" which is the random number the VBG Platform has generated for your caller (myuser01) to repeat in your IVR system.
Step 3 - Submit Sample to Transaction
Next, we'll assume that your IVR application has obtained the prompt (48163), played it for your caller (myuser01, perhaps using TTS), and has the recording of myuser01 repeating the prompt. So, you'll next want to submit the sample to the open/pending transaction in the VBG Platform. An example request using CURL follows:
We understand that IVR system developers will NOT be using local files. We used a local file reference here simply to demonstrate the basic sample submission process.
Now, returning to the JSON response, we can see a favorable result:
Step 4 - Check Transaction Information
As noted in our summary above, checking the status of your open Transaction is a best practice. Remember that a transaction equates to a session or a single engagement with a user. Within a typical IVR system, this can mean anywhere from 30 seconds to several minutes. Here is a sample CURL request:
In this case, we are not going to include the output, as it is essentially the same as Step #2. The main difference is that certain variables will update themselves over time. Monitoring transactions is particularly useful during an enrollment process, as several samples are typically gathered and evaluated in a loop.
Sample retries can come into play too. A user might not repeat the sample properly, or there could be too much background noise, or they may have scored too low. Monitoring transactions allows you to understand retries, and helps you to develop robust IVR logic, which can make the user experience smoother and more enjoyable.
Step 5 - Close the Transaction
Since we received a passing sample, it's time to close the transaction and record the status and score. An example request using CURL follows:
HTTP/1.1 200 OK
You may be asking "why do I need to record success and the score"? First of all, the VBG Platform is 100% stateless. This helps us with scaling and resiliency. But, it also echos the fact that we really don't know how all customers use the VBG Platform, or understand the intricacies of their businesses, risk exposure, etc.
For example, some customers have their own rule engines. They track user scores externally and ignore our pass/fail recommendation. Other customers prompt users twice for verification samples. VBG does not force anyone to adopt our models and techniques, so allowing the developer to close the transaction when ready offers the greatest amount of flexibility.
User Interaction Example
For this example, we'll use the same values we used above. However, we'll use an cloud-based IVR service provider, such as Twilio. And we'll also use VBG's Standard Dialogs, a collection of built-in IVR dialogs which fully manage the enrollment and verification process for every active use case we support. Here is the summary of the steps you'll take to verify myuser01:
-
Use GET for /users/{userid} API method to make sure userid exists and is active. This was covered previously, so please see the Custom Integration Example for details.
-
Use POST for /transactions API method to create a verification transaction. Response is then parsed for important information your application needs.
-
Use GET for /transactions/{transactionid} API method to get information about the verification process, along with other key measures. This was covered previously, so please see the Custom Integration Example for details.
-
ID. Transaction ID is always important. In this case, you can monitor the progress of the user within the hosted IVR system from any external/corporate application.
-
Phone Number. Since we are using a cloud-based IVR system, we provide back the appropriate phone number to call. You would pass this information to your end user from your own applications.
-
PIN. To allow multiple simultaneous inbound calls to the same number, we provide a temporary PIN for each user. When the IVR systems sees a particular PIN, we are able to tie it back to a particular transaction id and userid. Your application would give this to the end user as well (with the phone number).
NOTE: we'll only cover details for Step #2 below, and then will highlight the advantages of using this approach.
Step 2 - Create Verification Transaction
The syntax to create a verification transaction for myuser01 is identical to before. However, the VBG Platform has been configured differently and will respond differently. Here is the CURL request:
As you can see from the CURL request, the parameters for userid and type and calltype were provided. An example JSON response is as follows:
In this example, we've highlighted a couple new return values. Important notes are as follows:
Standard Plug-In Example
With VBG's Standard Plug-Ins and Adapters, there is very little, and often no, development needed. We use a combination of installed software, one of our active use cases, VBG Standard Dialogs, and provisioning management tools to provide multi-factor voice biometric authentication for many common scenarios. Our current plug-ins and adapters include:

RADIUS Server
Install our RADIUS Server Plug-In into your environment. We support both Windows and Linux servers. You can then easily configure Multi-Factor Authentication leveraging either outbound calls to a trusted phone number or "push" requests to our VBG Authenticator& trade' mobile app.
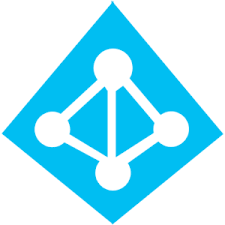
ADFS Adapter
Install our ADFS Adapter into your Windows Server environment. You can then easily configure Multi-Factor Authentication leveraging either outbound calls to a trusted phone number, WebRTC authentication via headsets, or "push" requests to our VBG Authenticator& trade' mobile app.
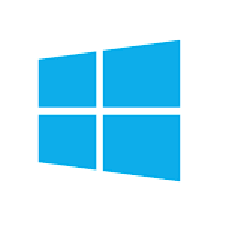
Windows LoginBeta
Install our Windows Credential Provider Plug-In onto your Windows computer. Then, at Windows startup, you can enable Multi-Factor Authentication leveraging either outbound calls to a trusted phone number or "push" requests to our VBG Authenticator™ mobile app.
Get Started Quickly
We make it easy to try voice biometrics with a free 60-day trial! See our Offerings page for additional details. All we require is a signed mutual NDA and a brief discovery session so that we can properly configure the VBG Platform for your needs.
As part of the trial, we'll give you access to our Visual Demo Center, which has an online API, working demos and code samples for all major platform functions, detailed documentation, and step-by-step "how-to" guides. We also give you access to our Dashboard, which provides real-time reporting on transactions, plus service logs to help troubleshoot your requests, and a variety of other ad-hoc reporting and analysis tools.
Finally, users in North America can also get free access to an IVR system and related inbound and outbound dialing support. We also include ASR support for active use cases.
Next Article in the Series
Do You Have Any Questions?
Please let us know how we can help you and we'll respond promptly!